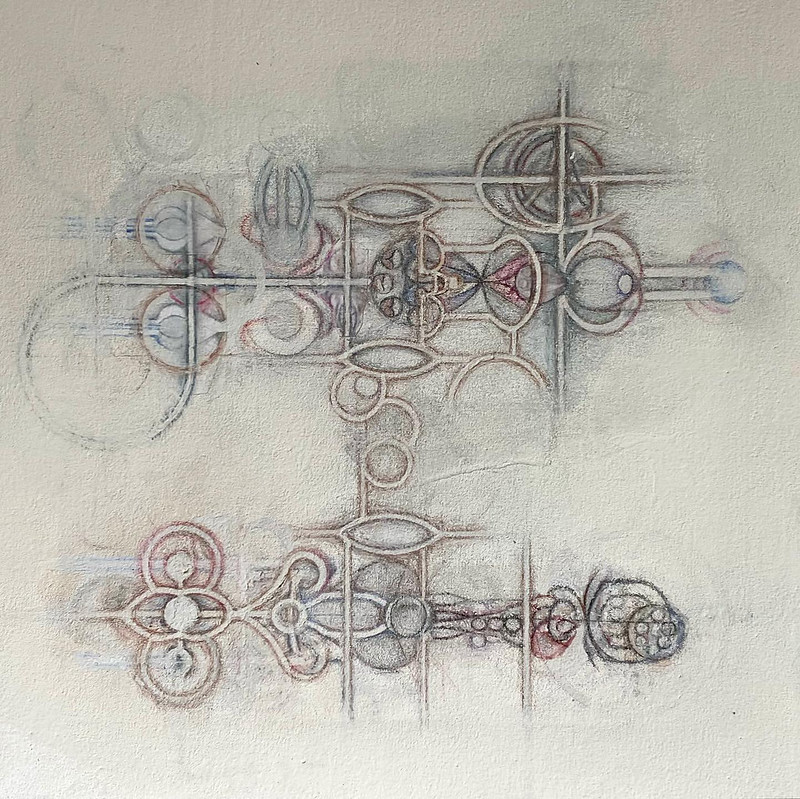
Mako is a template engine for Python. A Python program built to generate a HTML-formatted page can use Mako to populate data on that Web page, adding in custom data generated or imported by Python itself.
I use Make to create blog post pages for this web site. I have a template file for all the pages, and for each story I create a text file assigning the necessary metadata to Mako variables. The program itself creates other metadata entires, such as the date. When run, my program assembles the page from these elements, allowing me to file in the content after.
Mako is also used by Reddit, where it generates over a billion pages per month, according to Mako folks.
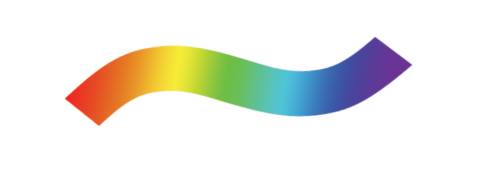
Here is how to use Mako to insert data, imported by Python, to a customized Web page.
To add this capability to your Python program, simply add a header in the program to call the Mako library:
from mako.template import Template
Template can be used to create a Python data structure of your text:
TheText = Template("hello world!") print(TheText.render())This data structure recognizes placeholders for variables you fill in. This is the format...
${VariableName}That can be embedded in the template:
from mako.template import Template ## Create a Variable SubjectName = "Walter" ## Set the Mako variable "ThisGuysName" within the text body... mytemplate = Template("Your name is ${ThisGuysName}!") #Assign the Mako variable to the Python variable in this format, MakoVariable=PythonVariable print(mytemplate.render(ThisGuysName=SubjectName))
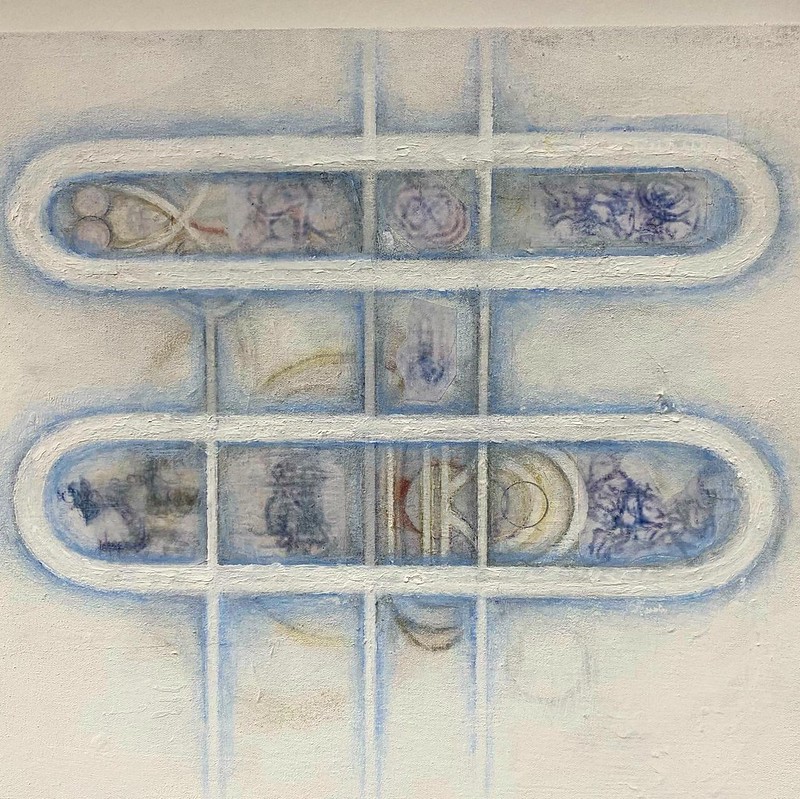
Template's "render" function creates the Python object, with the variables names, as well as a buffer to capture output.
Note: The Python variable name can be the same as the Mako variable name (I made the names separate for clarity). You still have to assign the Python variable to the Mako one i.e. "mytemplate.render(SubjectName=SubjectName)."
Loading Templates from Files: A template, with embedded variables, can be loaded from a file, using Template's 'filename' argument:
from mako.template import Template SubjectName = "Walter" mytemplate = Template(filename='MyTemplate.txt') #MyTemplate.txt contains the line "Your name is ${ThisGuysName}!" print(mytemplate.render(SubjectName=ThisGuysName))
A template, with the variables filled in, can then be written to a file:
from mako.template import Template #Set the template file to a variable mytemplate = Template(filename='MyTemplate.txt') #Fill in the variables FilledTemplate = mytemplate.render(SubjectName=ThisGuysName) #Create a file: FileName = "NamingNames.txt" f= open(FileName,"w+") #Write template data to the file created f.write(FilledTemplate) #Close file f.close()Art by Daffy Scanlan.